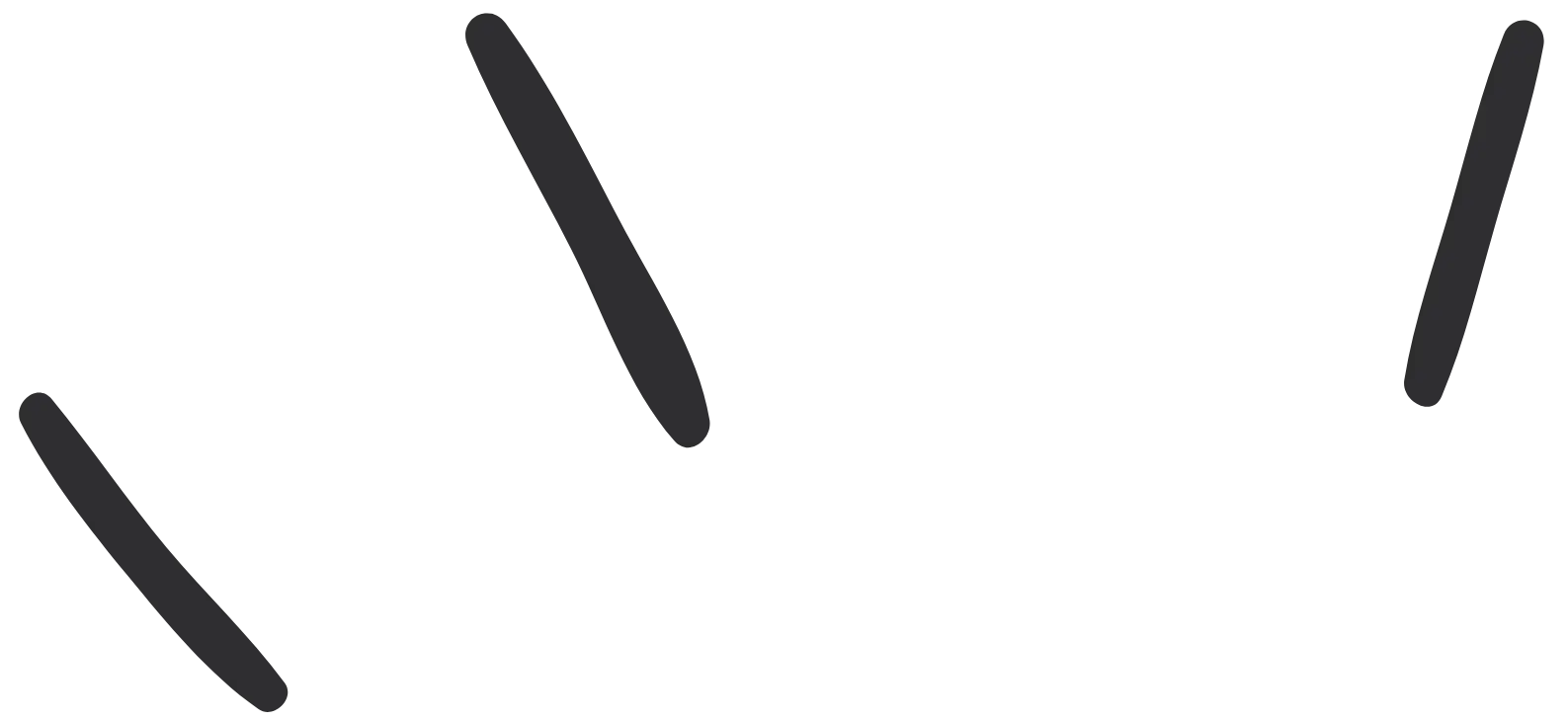
Form Builder Pesting with Livewire Volt
- Published: 06 Jul 2024 Updated: 07 Jul 2024
I’ll guide you on how to test your Form Builder using Livewire Volt with a class-based component.
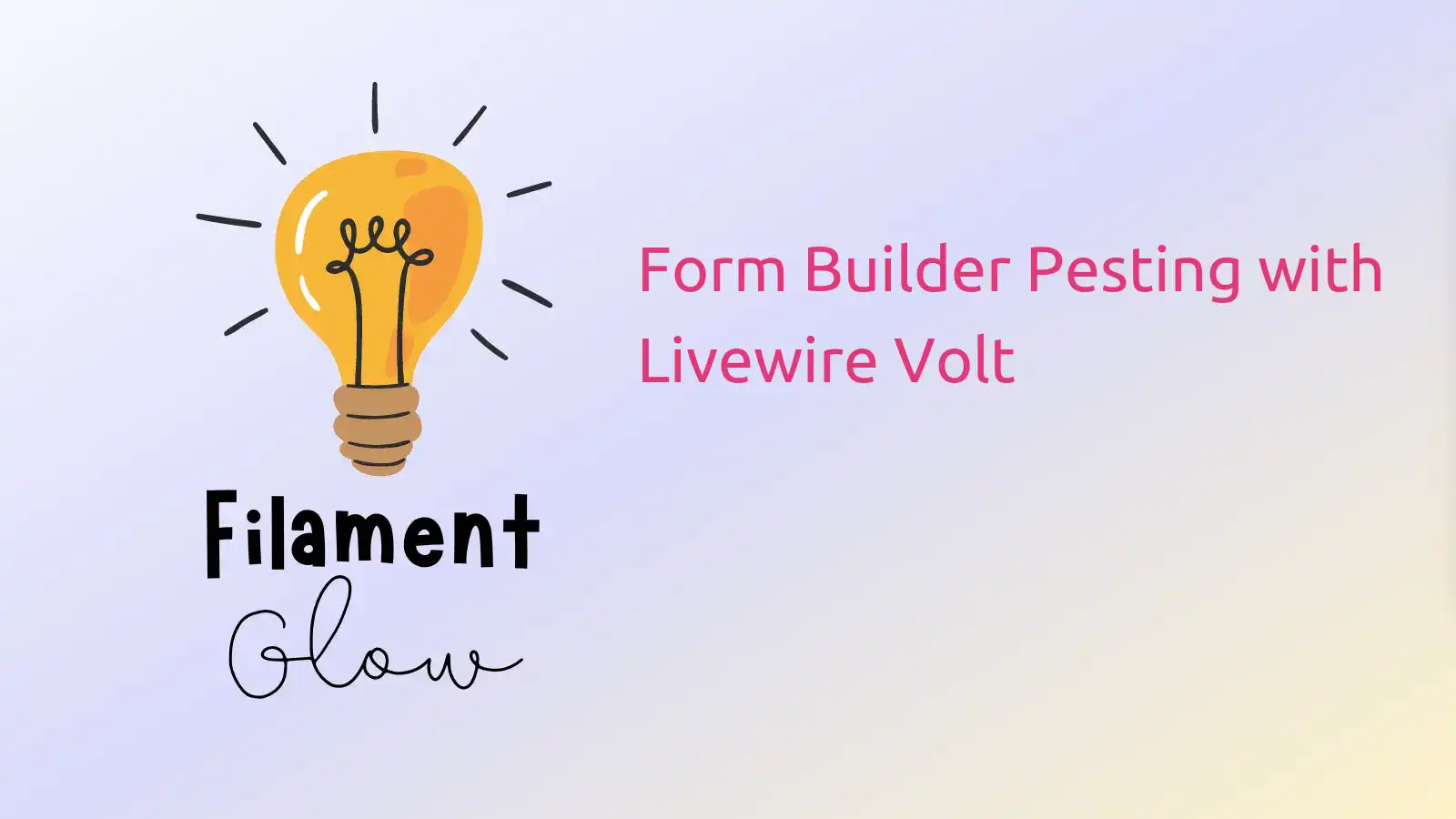
Introduction
Testing is a crucial phase in the software development life cycle, but it can be more challenging than expected.
I was curious about how to test with Form Builder and Volt together. So I googled it, and there are not so many topics that maybe it’s not. To help, I’ve created a blog to share my insights.
Today, I’ll guide you on how to test your Form Builder using Livewire Volt, a class-based component. In this project, my client's idea is for the customer to create an order from another e-commerce website, paste in the URL field, take a screenshot, and then make an order.
I will not cover the installation steps in this part of the guide. Let’s start with creating an order.
Creating Livewire Components
An Artisan command to create a new Volt component livewire/order/create.blade.php
.
A volt component creates only a blade file there is no Livewire/Order/Create.php
file.
That’s different from filament form builder testing docs.
Let’s see the code:
1<?php 2 3use Filament\Forms; 4use Filament\Forms\Form; 5use Livewire\Volt\Component; 6use Illuminate\Http\Response; 7use Illuminate\Support\Facades\Auth; 8use Filament\Forms\Contracts\HasForms; 9use Livewire\Attributes\{Layout, Title};10use Filament\Forms\Concerns\InteractsWithForms;11use Livewire\Features\SupportRedirects\Redirector;12 13new14#[Layout('layouts.app')]15#[Title('Make An Order')]16class extends Component implements HasForms {17 use InteractsWithForms;18 19 public ?array $data = [];20 21 public function mount(): void22 {23 $this->form->fill();24 }25 26 protected function form(Form $form): Form27 {28 return $form29 ->schema([30 Forms\Components\TextInput::make('url')31 ->url()32 ->prefixIcon('heroicon-m-globe-alt'),33 34 Forms\Components\FileUpload::make('screenshot')35 ->required()36 ->label(__('Screenshot'))37 ->directory('transaction-images')38 ->columnSpanFull(),39 ])40 ->statePath('data')41 ->model(App\Models\Order::class);42 }43 44 public function submit(): Redirector45 {46 abort_if(auth()->guest(), Response::HTTP_FORBIDDEN);47 48 $record = Auth::user()->orders()->create($this->form->getState());49 50 $this->form->model($record)->saveRelationships();51 52 return redirect()->route('dashboard');53 }54}; ?>55 56<div class="md:w-1/2 flex-auto md:pl-8 mt-5 md:mt-0">57 <form wire:submit.prevent="submit">58 <div>59 {{ $this->form }}60 </div>61 <div class="mt-5 flex justify-end">62 <x-primary-button class="ms-3">63 {{ __('Submit') }}64 </x-primary-button>65 </div>66 </form>67</div>
Creating a Pest
Firstly we need to check order create page is working or not.
tests/Feature/Order/CreateTest.php
1it('has order create page', function () {2 $this->actingAs(User::factory()->create());3 4 $response = $this->get('/order/create');5 6 $response->assertOk()->assertSeeVolt('order.create');7});
Ok. that’s working for me. Next test if the user is creating an order. Let’s see the code.
1test('user can order the product', function () { 2 $this->actingAs(User::factory()->create()); 3 4 $file = Illuminate\Http\UploadedFile::fake()->create('screenshot.jpg'); 5 6 livewire('order.create') 7 ->set('data.screenshot', $file) 8 ->fillForm([ 9 'data.url' => fake()->url(),10 'data.screenshot' => $file->hashName(),11 ])12 ->call('submit')13 ->assertHasNoFormErrors();14});
Awesome. Let’s break down what this code does step by step.
1. Creating a Fake File
1$file = Illuminate\Http\UploadedFile::fake()->create('screenshot.jpg');
The fake method is used to generate a fake file, in this case, a JPEG image. You can check here.
2. Interacting with the Livewire Component
1livewire('order.create')2 ->set('data.screenshot', $file)3 ->fillForm([4 'data.url' => fake()->url(),5 'data.screenshot' => $file->hashName(),6 ])7 ->call('submit')8 ->assertHasNoFormErrors();
Here, we are using the Livewire testing utilities to interact with the order.create
component:
You can use blade view directly in the livewire plugins livewire(‘order.create’)
We use the set method to assign the fake file to the screenshot properties.
The call(‘submit’)
method simulates the form submission.
- Finally, assertHasNoFormErrors
ensures that the form submission did not return any validation errors, confirming the process was successful.
Conclusion
This test case demonstrates how to simulate a user ordering a product in a Laravel application using Livewire Volt. It covers user authentication, file uploads, and form submissions, ensuring that your component behaves as expected under these conditions. By writing thorough tests like this one, you can maintain high-quality code and provide a robust user experience. Thank you so much.
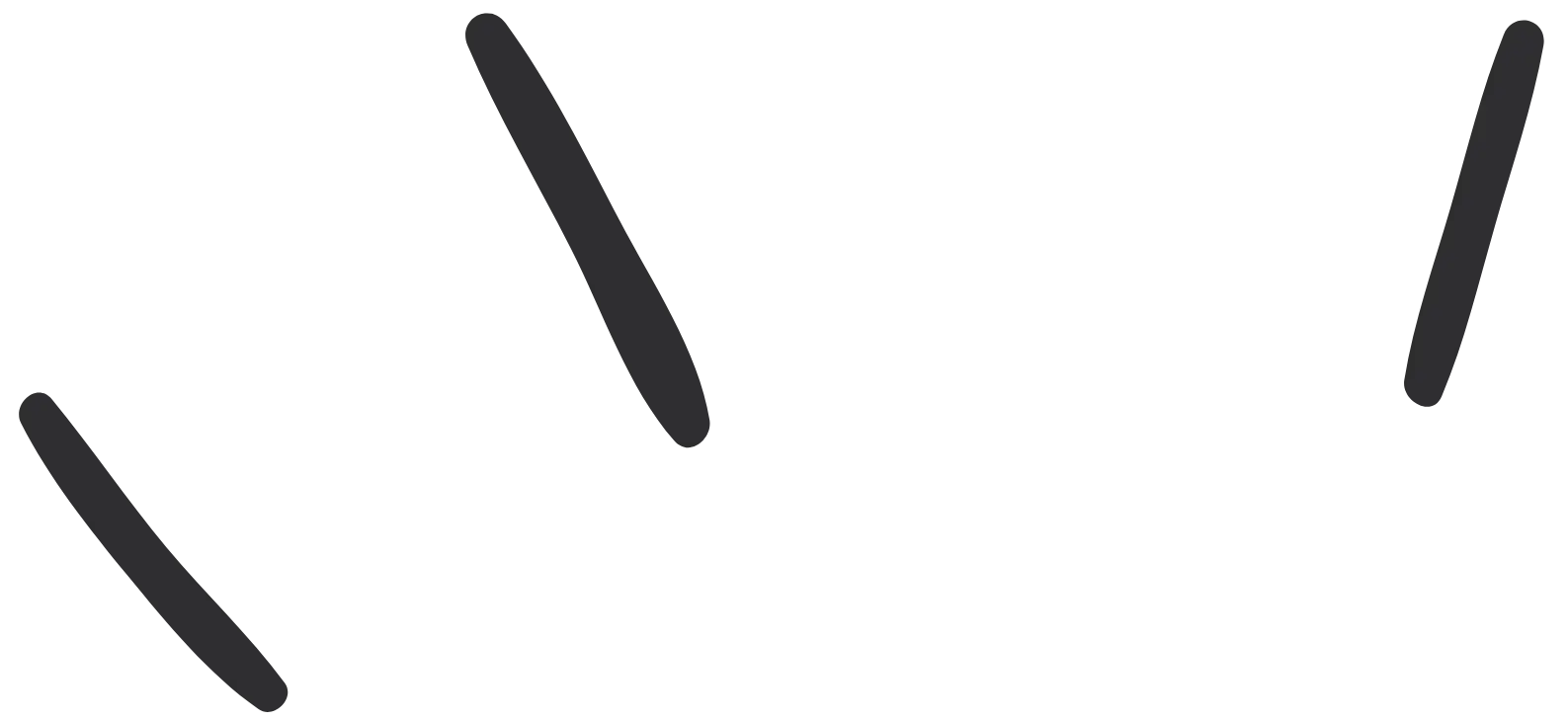
Related Tricks:
I’ll guide you on how to test your Form Builder using Livewire Volt with a class-based component.
how to use a resource with multiple models
Usability: Knowing whether or not the record was saved.
How to Apply Authorization on Create Option Action for Select Field
get translatable attribute in a relationships