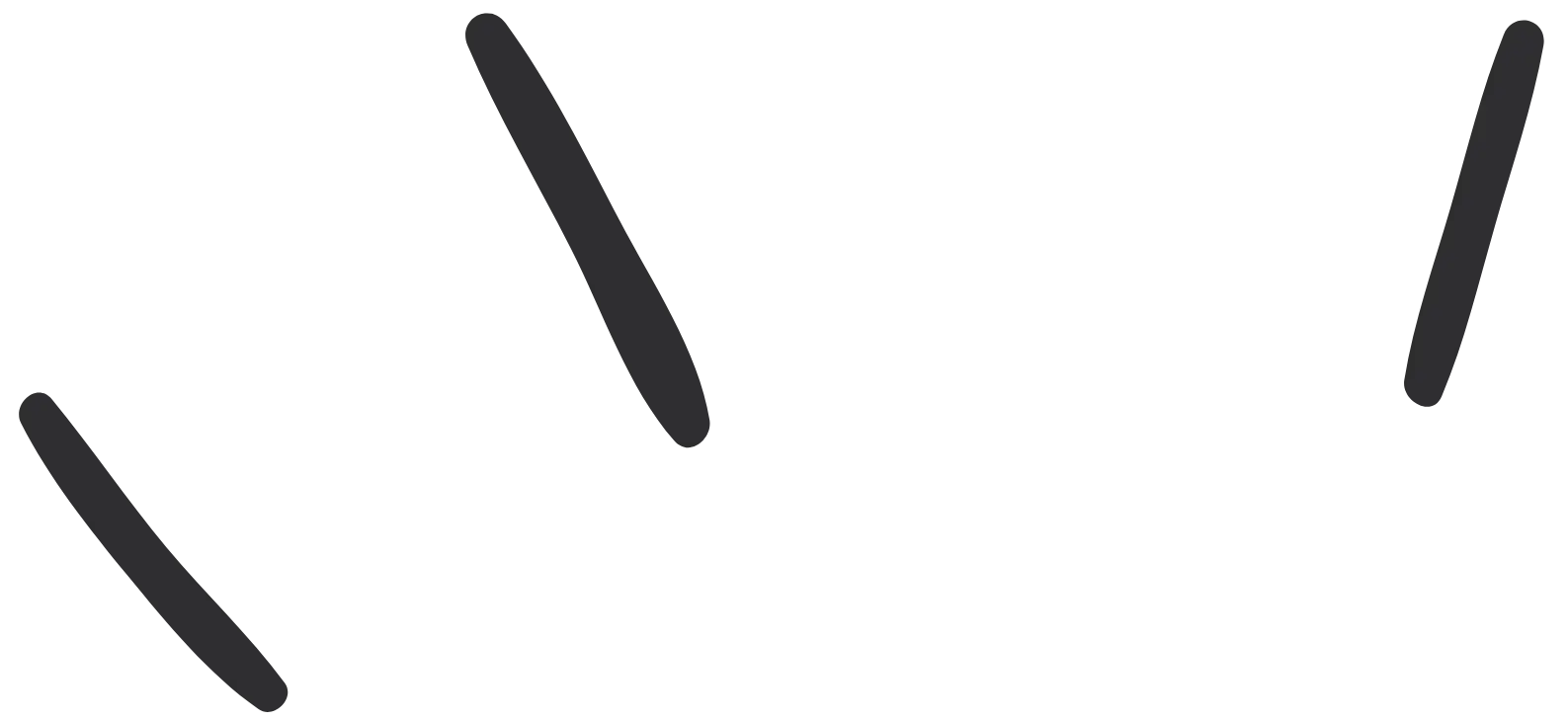
Disabling the cancel button on the filament editing form
- Published: 20 Sep 2024 Updated: 23 Sep 2024
Usability: Knowing whether or not the record was saved.
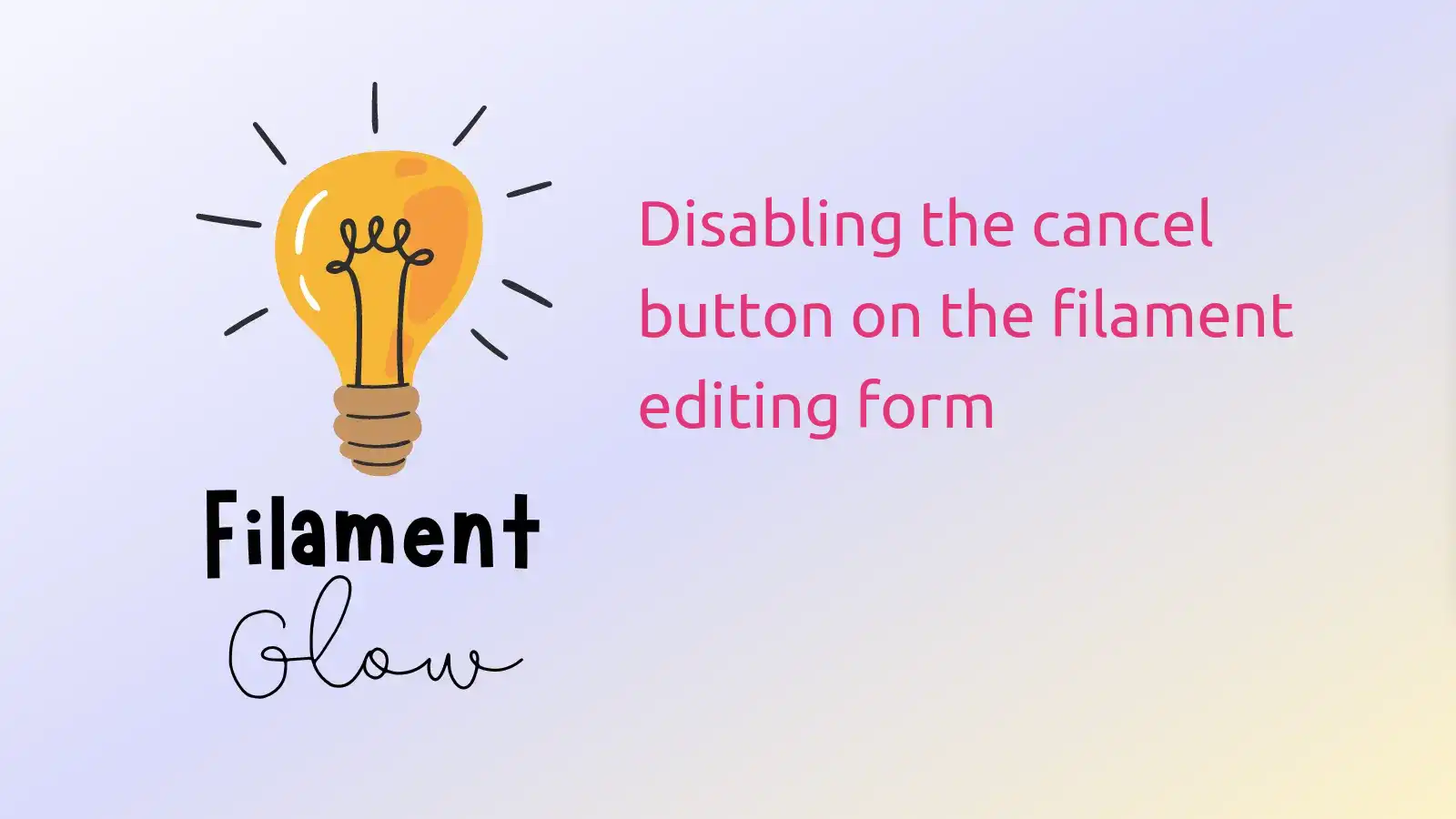
1<?php 2 3namespace App\Filament\Resources\ContactResource\Pages; 4 5use App\Filament\Resources\ContactResource; 6use Filament\Actions; 7use Filament\Resources\Pages\EditRecord; 8use Filament\Actions\Action; 9 10class EditContact extends EditRecord11{12 protected static string $resource = ContactResource::class;13 14 protected bool $editing = false;15 16 public function mount(int|string $record): void17 {18 $this->editing = request()->has('editing');19 20 parent::mount($record);21 }22 23 protected function getCancelFormAction(): Action24 {25 return Action::make('cancel')26 ->disabled($this->editing)27 ->label(__('filament-panels::resources/pages/edit-record.form.actions.cancel.label'))28 ->url($this->getResource()::getUrl('index'))29 ->color('gray');30 }31 32 protected function getHeaderActions(): array33 {34 return [35 Actions\DeleteAction::make(),36 ];37 }38 39 protected function getRedirectUrl(): string40 {41 return $this->getResource()::getUrl('edit', ['record' => $this->getRecord()]);42 }43}
1// Resource Page -> ContactResource.php 2Tables\Actions\Action::make('edit') 3 ->label('Editar') 4 ->icon('heroicon-o-pencil') 5 ->url(function ($record) { 6 $modelClass = static::getModel(); 7 $modelInstance = new $modelClass(); 8 $tableName = $modelInstance->getTable(); 9 $customEditRoute = route(10 "filament.admin.resources.{$tableName}.edit",11 [12 $record,13 'editing' => 114 ]15 );16 return $customEditRoute;17 }),
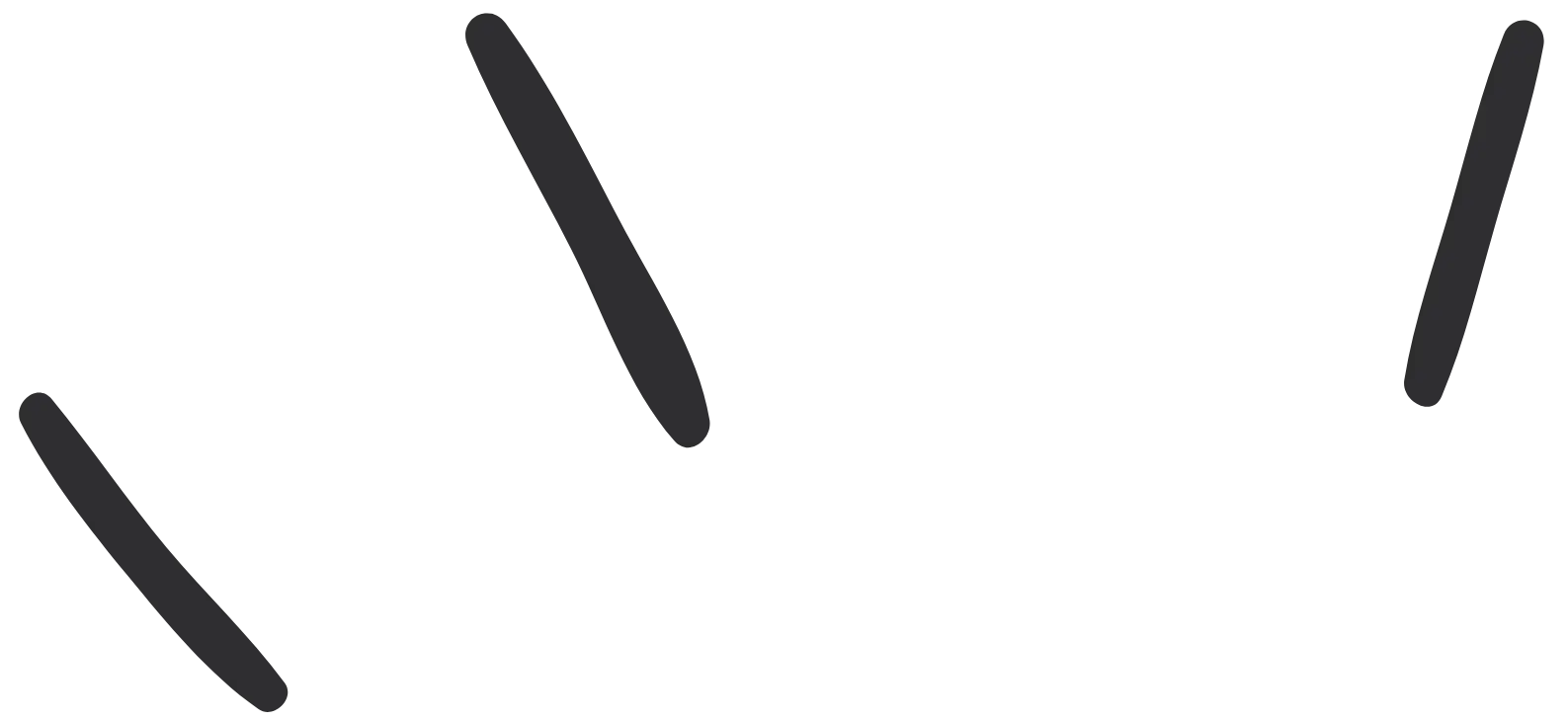
Related Tricks:
get translatable attribute in a relationships
Usability: Knowing whether or not the record was saved.
I’ll guide you on how to test your Form Builder using Livewire Volt with a class-based component.
How to Apply Authorization on Create Option Action for Select Field
Sometimes, when we need to test our application locally, we always have to fill forms manually. Let's take the Filament approach and achieve this with more accurate data.