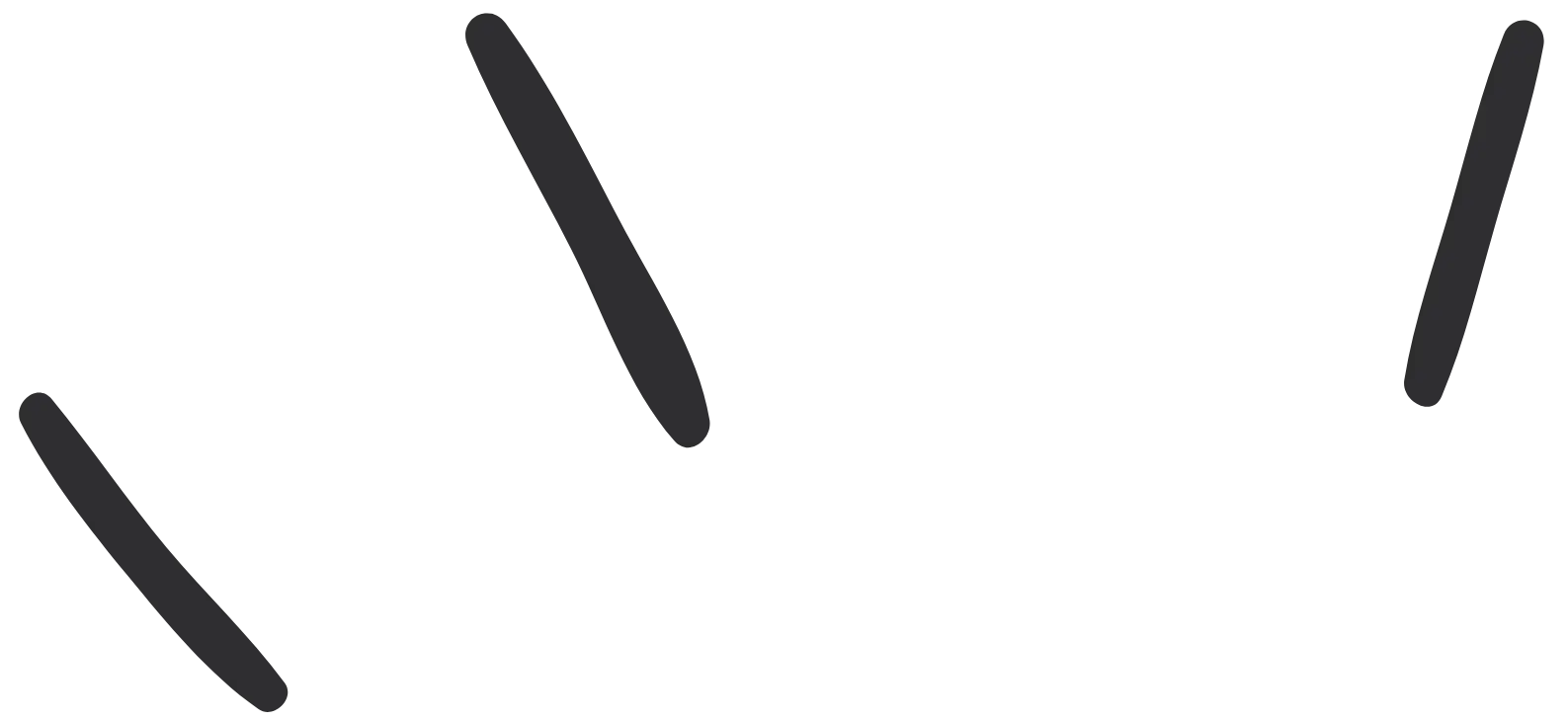
Filament form with factory data
- Published: 24 Oct 2024 Updated: 24 Oct 2024
Sometimes, when we need to test our application locally, we always have to fill forms manually. Let's take the Filament approach and achieve this with more accurate data.
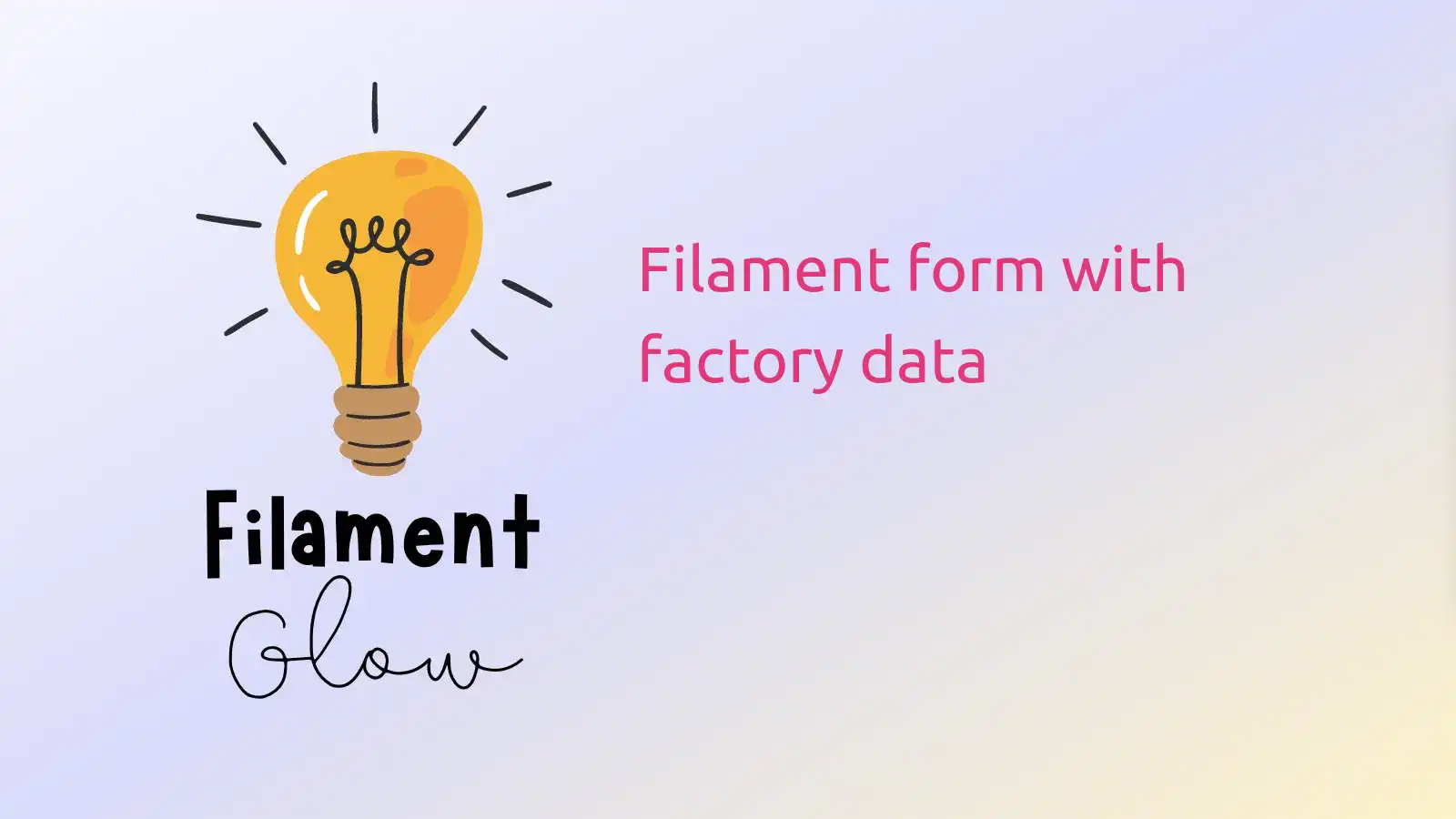
Prepare the Factory definition
1class PostFactory extends Factory 2{ 3 public function definition(): array 4 { 5 $title = $this->faker->sentence; 6 7 return [ 8 'title' => $title, 9 'slug' => str($title)->slug()->toString(),10 'excerpt' => $this->faker->sentences(3, true),11 'body' => $this->faker->sentences(10, true),12 ];13 }14}
Fill the Form Action
1<?php 2 3namespace App\Filament\Resources\PostResource\Pages; 4 5use App\Filament\Resources\PostResource; 6use App\Models\Post; 7use Filament\Actions\Action; 8use Filament\Resources\Pages\CreateRecord; 9 10class CreatePost extends CreateRecord11{12 protected static string $resource = PostResource::class;13 14 protected function getHeaderActions(): array15 {16 return [17 Action::make('form-fake-filler')18 ->label('Fill Form')19 ->icon('heroicon-o-sparkles')20 ->color('info')21 ->action(function(self $livewire): void {22 $data = Post::factory()->make()->toArray();23 24 $livewire->form->fill($data);25 }),26 ];27 }28}
Let's show this button only when we are in development mode. To achieve this, let's add a condition to our action definition:
1//... 2protected function getHeaderActions(): array 3{ 4 return [ 5 Action::make('form-fake-filler') 6 //... 7 ->action(function(self $livewire): void { 8 $data = Post::factory()->make()->toArray(); 9 10 $livewire->form->fill($data);11 })12 ->visible(fn () => app()->environment('local'))13 ];14}15//...
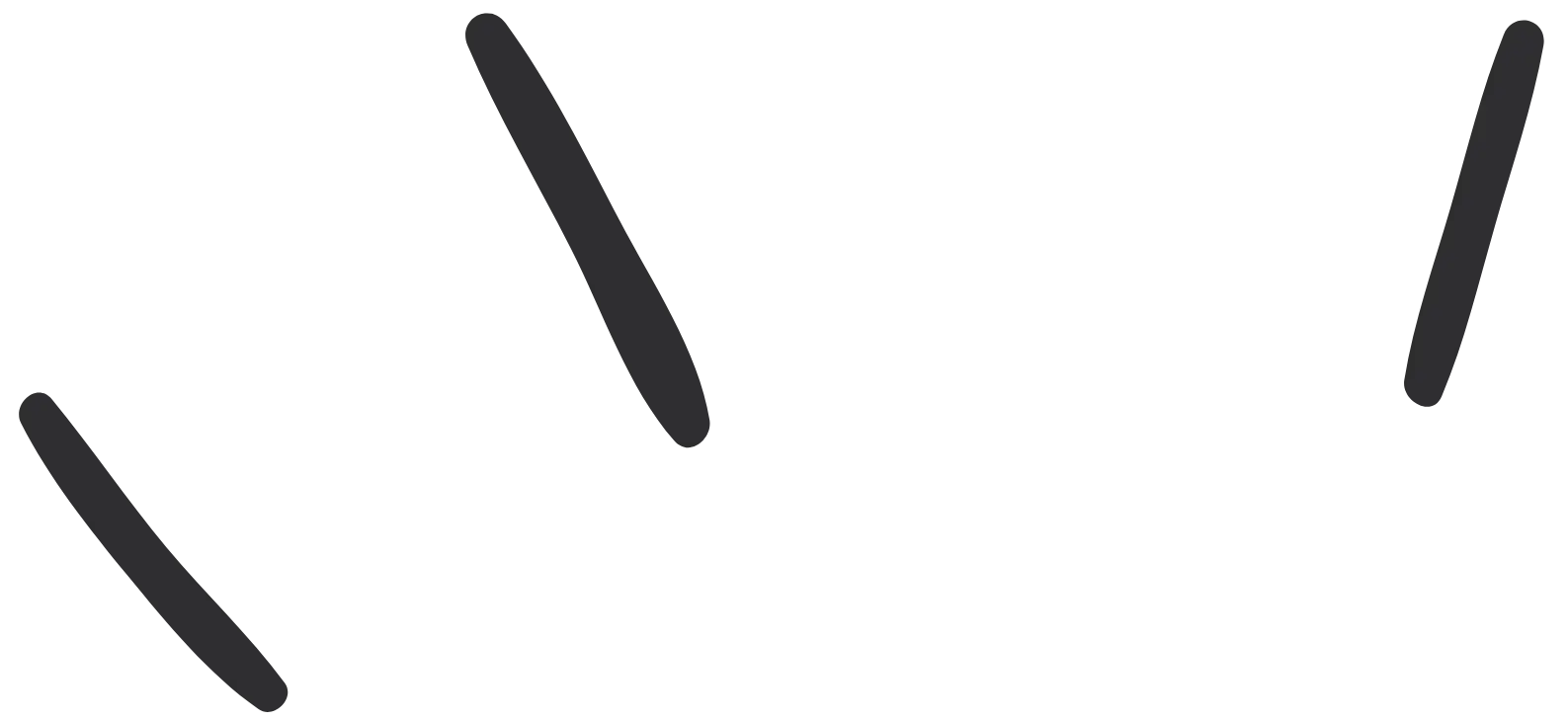
Related Tricks:
Let Users to Select All Options with a Simple Hint Action
how to use a resource with multiple models
I’ll guide you on how to test your Form Builder using Livewire Volt with a class-based component.
Translating components can often be a repetitive task, Fortunately, there's a neat trick to automate this process, making your components instantly translatable.
toggle the visibility of an action when hover the component